Normal view
-
- C# MVVM Toolkit DemoThis article and the demo are about getting started using the MVVM Toolkit and some self-created interfaces / services for MessageBox and some dialogs.
-
- How do you format the text displayed by geom_text_contour?MWE: library(metR) heat_palette = c("darkred", "red", "yellow", "#00A600", "darkgreen") heatmap_of_percentage <- function(percent) { # display <- sprintf("%2f%%", {{percent}}) # ^^ If I uncomment this, I get a bug c( geom_raster(aes(fill = {{percent}})), geom_contour(aes(z = {{percent}}), color = "black"), geom_text_contour(aes(z = {{percent}}), color = "black", stroke = 0.2), scale_fill_gradientn(limits = c(0, 100), colours = heat_palette) ) } df <- expan
How do you format the text displayed by geom_text_contour?
MWE:
library(metR)
heat_palette = c("darkred", "red", "yellow", "#00A600", "darkgreen")
heatmap_of_percentage <- function(percent) {
# display <- sprintf("%2f%%", {{percent}})
# ^^ If I uncomment this, I get a bug
c(
geom_raster(aes(fill = {{percent}})),
geom_contour(aes(z = {{percent}}), color = "black"),
geom_text_contour(aes(z = {{percent}}), color = "black", stroke = 0.2),
scale_fill_gradientn(limits = c(0, 100), colours = heat_palette)
)
}
df <- expand.grid(x = 1:100, y = 1:100)
df$Z <- df$x * df$y / 100
ggplot(df, aes(x = x, y = y)) +
heatmap_of_percentage(percent = Z)
produces
I would like to modify this so that the contours are labelled 10%, 30%, etc.. How can I do this, only modifying heatmap_of_percentage
(i.e. not adding an extra column in the calling code)?
-
- Jawohl answer when someone knocks at the door โ german.stackexchange.comIn the first episode of the TV series "Kleo", a Stasi colonel says "Jawohl" to allow people to enter his office when they knock at the door. The person knocking is unknown and in ...
Jawohl answer when someone knocks at the door โ german.stackexchange.com
-
- A Wave of AI Tools Is Set to Transform Work MeetingsAn AI-powered wearable from startup Limitless promises to make meetings more productive. Itโs the beginning of a wider transformation of human interactions.
A Wave of AI Tools Is Set to Transform Work Meetings
-
- Thomson Reuters Lays Out Plan To Provide CoCounsel AI Assistant Across Every Professional Vertical It ServesThomson Reuters, continuing to build on its acquisition last June of Casetext and its CoCounsel generative AI legal assistant for a whopping $650 million cash, today disclosed plans to deploy CoCounsel as a single and continuous AI assistant across its entire portfolio of products spanning every professional its serves in legal, tax, risk and fraud, [โฆ]
Thomson Reuters Lays Out Plan To Provide CoCounsel AI Assistant Across Every Professional Vertical It Serves
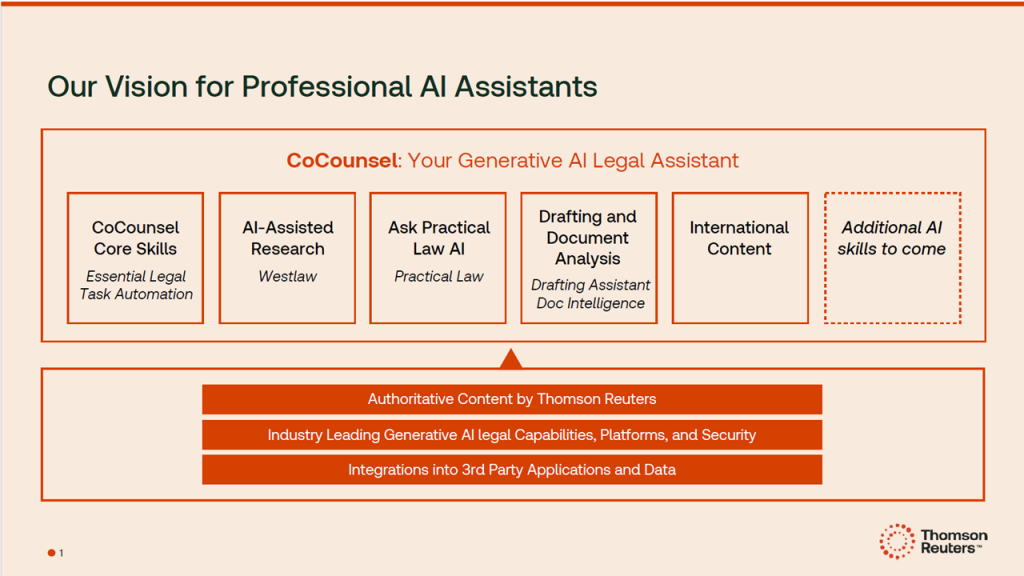
-
- should EF dbContext be created on every transactionI'm trying to figure out the best way to manage the DbContext. I've seen code samples that don't dispose and I've seen people say that that is a bad idea. Is it appropriate for me to do something like below? Also, should I put every transaction, including reads, in a new DbContext? This might be another question, but is the part about the EntityState necessary? public abstract class GenericRepository<T> where T : EntityData { protected MyDbContext Context { get { return ne
should EF dbContext be created on every transaction
I'm trying to figure out the best way to manage the DbContext. I've seen code samples that don't dispose and I've seen people say that that is a bad idea. Is it appropriate for me to do something like below? Also, should I put every transaction, including reads, in a new DbContext? This might be another question, but is the part about the EntityState necessary?
public abstract class GenericRepository<T> where T : EntityData
{
protected MyDbContext Context
{
get { return new MyDbContext(); }
}
public T Save(T obj)
{
T item;
using (var context = Context)
{
var set = context.Set<T>();
if (String.IsNullOrEmpty(obj.Id))
item = set.Add(obj);
else
{
item = set.Find(obj.Id);
item = obj;
}
// taken from another code sample
var entry = context.Entry(item);
if (entry.State == EntityState.Detached)
{
//Need to set modified so any detached entities are updated
// otherwise they won't be sent across to the db.
// Since it would've been outside the context, change tracking
//wouldn't have occurred anyways so we have no idea about its state - save it!
set.Attach(item);
context.Entry(item).State = EntityState.Modified;
}
context.SaveChanges();
}
return item;
}
}
EDIT
I also have an extended class that implements this function below. The context is not being wrapped in a using statement in this query, so I'm a little suspicious of my code.
public IQueryable<T> FindByAccountId(string accountId)
{
return from item in Context.Set<T>()
let user = UserRepository.FindByAccountId(accountId).FirstOrDefault()
where item.UserId == user.Id
select item;
}
-
- Tech Leaders Once Cried for AI Regulation. Now the Message Is โSlow DownโAny dreams of a sweeping AI bill out of Congress are basically a hallucination.
Tech Leaders Once Cried for AI Regulation. Now the Message Is โSlow Downโ
-
- how tagging index in rst fileI have a file structure that I want to publish docomentation to confluence using sphinx. But there is a problem, I don't understand how to add a link to the main page structure Home page ======================== Navigation ======================== .. toctree:: :maxdepth: 3 index first second third anything What tags do I need to add in order for sphinx to automatically generate a link to the index conf.py structure extensions = [ 'sphinxcontrib.confluencebuilder', ]
how tagging index in rst file
I have a file structure that I want to publish docomentation to confluence using sphinx. But there is a problem, I don't understand how to add a link to the main page
structure
Home page
========================
Navigation
========================
.. toctree::
:maxdepth: 3
index
first
second
third
anything
What tags do I need to add in order for sphinx to automatically generate a link to the index
conf.py
structure
extensions = [
'sphinxcontrib.confluencebuilder',
]
confluence_publish = True
confluence_space_key = 'username'
confluence_server_url = 'confluence.server.com'
confluence_page_hierarchy = True
confluence_page_generation_notice = True
confluence_prev_next_buttons_location = 'top'
confluence_server_user = 'username'
confluence_server_pass = 'password'
-
- in Flutter how to Dissmiss the context menu of SPdfViewer when the specific text is selected?enter image description herei would like to hide this default context menu of SfPdfViewer which provided by 'package:syncfusion_flutter_pdfviewer/pdfviewer.dart' and don't want to be showed when the user select a specific word. Anyone has any idea how to do it ? a solitoun of the issue or problem that iam facing now
in Flutter how to Dissmiss the context menu of SPdfViewer when the specific text is selected?
enter image description herei would like to hide this default context menu of SfPdfViewer which provided by 'package:syncfusion_flutter_pdfviewer/pdfviewer.dart' and don't want to be showed when the user select a specific word. Anyone has any idea how to do it ?
a solitoun of the issue or problem that iam facing now
-
- ". . . those who feel able to answer this call for help, have a strong cup of coffee and be on your way." โ ell.stackexchange.com(From A Terrible Kindness by Jo Browning Wroe) Part I, Aberfan, chapter 2 (The end of the dinner party) "Please share with gathered institute members." . . . "Embalmers needed urgently ...
". . . those who feel able to answer this call for help, have a strong cup of coffee and be on your way." โ ell.stackexchange.com
-
- This AI Startup Wants You to Talk to Houses, Cars, and FactoriesArchetype builds AI models that act as a translation layer between humans and complex sensors, using plain language to help people understand whatโs happening in a building, car, or human body
This AI Startup Wants You to Talk to Houses, Cars, and Factories
-
- Excel txt file import (specific columns) into a table with VBAI found this forum while I was trying to sort out my VBA code. I only have very little experience in VBA programming so far. That is why I have researched since two days for a solution for my problem, without success. However, I am very interested in impoving my programming skillsa and I hope you can help me to do so. Basically I would like to import a TXT file into the active Excel workbook, sheet named "DataImport" at the end of the table named "TblDataImport". The TXT file consists of 13 co
Excel txt file import (specific columns) into a table with VBA
I found this forum while I was trying to sort out my VBA code. I only have very little experience in VBA programming so far. That is why I have researched since two days for a solution for my problem, without success. However, I am very interested in impoving my programming skillsa and I hope you can help me to do so.
Basically I would like to import a TXT file into the active Excel workbook, sheet named "DataImport" at the end of the table named "TblDataImport".
The TXT file consists of 13 columns which are separated by tabs:
- row: CompanyName
- row: Date Name CustomerGroup CustomerNo SalesOrder ItemNumber ItemGroup LineStatus Quantity Price Discount DiscountPercentage NetAmount
- row: All corresponding values...
I dont't need to import the first and the second row since the table where I would like to import the data to aready exists including headings. Also I only need 7 out of the 13 columns, those that are formated in bold. It would be perfect if the user could choose the text file using an open file dialogue.
If I open the TXT file manually and paste its content into Excel, it is already included
in the right columns. So there should not be any pitfalls with regard to formatting.
I am curious about the solutions you come up with.
With the macro recorder I can only fulfil some of my conditions:
Sub DataImport()
' DataImport Makro
Sheets("DataImport").Select
With ActiveSheet.QueryTables.Add(Connection:= _
"TEXT;C:\Users\Sales.txt", Destination:=Range _
("$A$1"))
.Name = "AxaptaSales"
.FieldNames = True
.RowNumbers = False
.FillAdjacentFormulas = False
.PreserveFormatting = True
.RefreshOnFileOpen = False
.RefreshStyle = xlInsertDeleteCells
.SavePassword = False
.SaveData = True
.AdjustColumnWidth = True
.RefreshPeriod = 0
.TextFilePromptOnRefresh = False
.TextFilePlatform = 850
.TextFileStartRow = 1
.TextFileParseType = xlDelimited
.TextFileTextQualifier = xlTextQualifierDoubleQuote
.TextFileConsecutiveDelimiter = False
.TextFileTabDelimiter = True
.TextFileSemicolonDelimiter = False
.TextFileCommaDelimiter = False
.TextFileSpaceDelimiter = False
.TextFileColumnDataTypes = Array(4, 1, 9, 9, 1, 9, 9, 9, 1, 1, 1, 9, 1)
.TextFileTrailingMinusNumbers = True
.Refresh BackgroundQuery:=False
End With
End Sub
However this code only pastes all columns into cell A1 (not at the end of the table). Also it includes the first and second row as well as the columns I don't need.
-
- Why does getText() return an empty string even if the text field is not empty?For context I am creating a button wherein when player presses it, it gets the text in the text area and does its thing /** * Creates and displays the name input Confirm button * @param nameInputActionListener The action to be done when confirm button is pressed */ public void createAndDisplay_nameInputConfirmButton(ActionListener nameInputActionListener) { this.nameInputConfirmButton = new JButton("Confirm"); this.nameInputConfirmButton.addActionListener(nameInputActionListener);
Why does getText() return an empty string even if the text field is not empty?
For context I am creating a button wherein when player presses it, it gets the text in the text area and does its thing
/**
* Creates and displays the name input Confirm button
* @param nameInputActionListener The action to be done when confirm button is pressed
*/
public void createAndDisplay_nameInputConfirmButton(ActionListener nameInputActionListener)
{
this.nameInputConfirmButton = new JButton("Confirm");
this.nameInputConfirmButton.addActionListener(nameInputActionListener);
this.nameInputConfirmButton.setBounds(1250, 340, 100, 50);
this.characterCreationScreenFrame.add(this.nameInputConfirmButton);
}
this.nameInputArea = new JTextField(40);
this.nameInputArea.setBounds(950, 350, 250, 30);
this.nameInputArea.setToolTipText("Enter Name here!");
this.characterCreationScreenFrame.add(this.nameInputArea);
/**
* @return The text inputted by player in the name input text area
*/
public String getNameInputtedByPlayer()
{
return this.nameInputArea.getText();
}
I tried doing the same concept of implementation as taught in a YouTube video and it works, so I don't understand why this wouldn't. The main issue is getText()
does not retrieve anything.
here is the action listener of it
/** * Creates the action associated with the Name Input button. Moreover, it temporarily hides the character creation buttons. */ private void create_ActionFor_NameInputButton() { // NOTE! dinispose NOT HIDE kasi para kapag player goes back to charac screen gagawa ulit new gui para updated yung current charac details characterCreationScreenView.hide_CharacterCreationScreenChoicesList(); characterCreationScreenView.createAndDisplay_NameInput();
// ACTION TO BE DONE KAPAG NAGCONFIRM SI PPLAYER AFTER INPUTTING A NAME
ActionListener nameInput_ActionListener = new ActionListener()
{
@Override
public void actionPerformed(ActionEvent nameInput)
{
// gets the text na nilagay sa text area and checks if it valid (at least > 1) IF OO TRIM NAME IN CASE EXCEEDING 25
if(characterCreationScreenModel.checkIfNameInputIsValid(characterCreationScreenView.getNameInputtedByPlayer()) == true)
{
String validatedName = characterCreationScreenModel.remove_CharactersExceeding25(characterCreationScreenView.getNameInputtedByPlayer());
characterCreationScreenModel.setPlayerName(validatedName);
characterCreationScreenView.hide_DisplayNameInput();
characterCreationScreenView.unhide_CharacterCreationScreenChoicesList();
characterCreationScreenView.createAndDisplay_CurrentCharacterDetailsLabels();
}
else
{
characterCreationScreenView.display_NameInputErrorMessage();
System.out.println("" +characterCreationScreenView.getNameInputtedByPlayer().length());
}
}
};
//characterCreationScreenView.createAndDisplay_nameInputConfirmButton(nameInput_ActionListener);
characterCreationScreenView.createAndDisplay_nameInputConfirmButton(nameInput_ActionListener);
} here is the example output [example][1]
-
- Hereโs Proof the AI Boom Is Real: More People Are Tapping ChatGPT at WorkDespite recent warnings that generative AI is overhyped, new data from Pew Research Center shows a rapid increase in the number of people who have used ChatGPT at work.
Hereโs Proof the AI Boom Is Real: More People Are Tapping ChatGPT at Work
-
- TypeError: Cannot destructure property 'posts' of '(0 , react__WEBPACK_IMPORTED_MODULE_1__.useContext)(...)' as it is undefinedI am using the context in nextjs 14 for managing the state. This is the provider at top level page.js When using dynamic routing, slug(page.js), I am not able to get the context values. Can anyone help in this on how to access context values here in slug. Can anyone provide a solution or fix to this.
TypeError: Cannot destructure property 'posts' of '(0 , react__WEBPACK_IMPORTED_MODULE_1__.useContext)(...)' as it is undefined
I am using the context in nextjs 14 for managing the state. This is the provider at top level page.js
When using dynamic routing, slug(page.js), I am not able to get the context values. Can anyone help in this on how to access context values here in slug.
Can anyone provide a solution or fix to this.
-
- Fast Companyโs List of Worldโs 606 Most Innovative Companies Includes Four from Legal TechFast Company is out with its annual ranking of the worldโs most innovative companies, and of the 606 companies that made the list, just four are from legal tech. โThe 606 organizations that we honor as Fast Companyโs Most Innovative Companies of 2024 have met our high bar for demonstrating innovation and the impact of [โฆ]
Fast Companyโs List of Worldโs 606 Most Innovative Companies Includes Four from Legal Tech
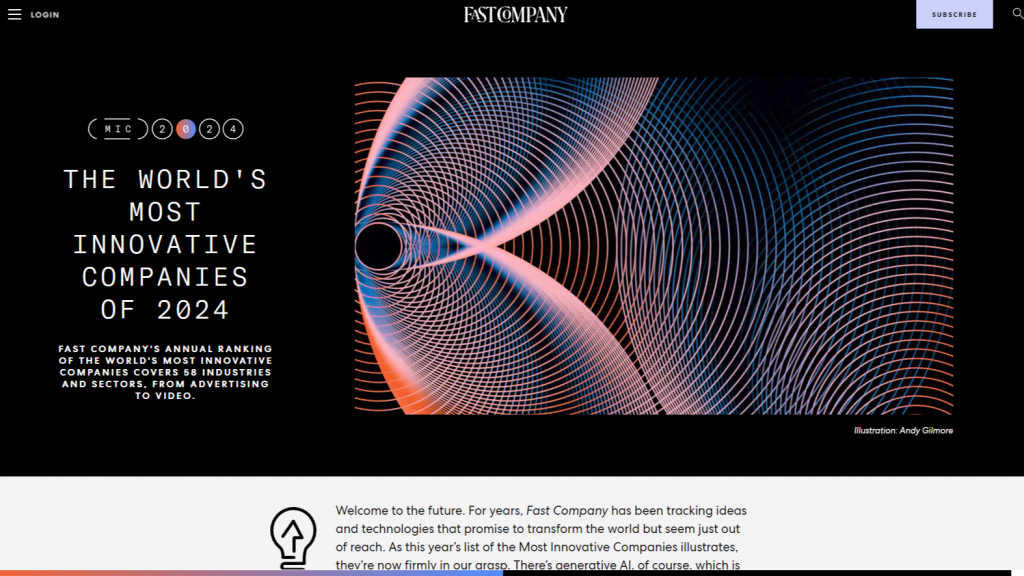
-
- Why aren't "Bottom" and "Distance to deep water" the same in this context? โ ell.stackexchange.comIn Mission Impossible - Dead Reckoning Part One (2023), Captain speaks to Officer in a submarine: Captain: Officer of the Deck, ship status? Officer: Ship's depth, 50 meters. Bottom, 72 meters. ...
Why aren't "Bottom" and "Distance to deep water" the same in this context? โ ell.stackexchange.com
-
- Why "Bottom" and "Distance to deep water" the aren't same in this context? โ ell.stackexchange.comIn Mission Impossible - Dead Reckoning Part One (2023), Captain speaks to Officer in a submarine: Captain: Officer of the Deck, ship status? Officer: Ship's depth, 50 meters. Bottom, 72 meters. ...
Why "Bottom" and "Distance to deep water" the aren't same in this context? โ ell.stackexchange.com
-
- Trying to add color coding to the title of ggplotlyI am trying to produce a graph where the title has color coded text to remove the need for a legend. I have no problem doing this in ggplot2 using the ggtext package and ggtext::element_markdown(). However, when I try to then layer on top ggplotly, the color disappears. I am wondering if there is any way to combine the color functionality with ggplotly? Here is a sample reproducible example using the mtcars dataset. library(ggplot2) library(dplyr) library(plotly) library(ggtext) mtcolors<-c(
Trying to add color coding to the title of ggplotly
I am trying to produce a graph where the title has color coded text to remove the need for a legend. I have no problem doing this in ggplot2
using the ggtext
package and ggtext::element_markdown()
.
However, when I try to then layer on top ggplotly
, the color disappears.
I am wondering if there is any way to combine the color functionality with ggplotly
?
Here is a sample reproducible example using the mtcars
dataset.
library(ggplot2)
library(dplyr)
library(plotly)
library(ggtext)
mtcolors<-c("springgreen4", "darkorange3", "purple")
names(mtcolors)<-c("4 cyl", "6 cyl", "8 cyl")
mt_title_text <- glue::glue(
'MPG vs HP -',
'<span style = "color:{mtcolors["4 cyl"]}">**4 cyl**</span>',
'vs.',
'<span style = "color:{mtcolors["6 cyl"]}">**6 cyl**</span>',
'vs.',
'<span style = "color:{mtcolors["8 cyl"]}">**8 cyl**</span>',
.sep = ' '
)
mtcars |>
mutate(
cyl = as.factor(cyl)
) |>
ggplot(aes(x=mpg, y=hp, color=cyl)) +
geom_point(size=3) +
theme_bw() +
scale_color_manual(values=c("springgreen4", "darkorange3", "purple")) +
labs(
title = mt_title_text
) +
theme(
plot.title = ggtext::element_markdown(),
panel.grid.minor = element_blank(),
legend.position = 'none'
)
This produces the following graph:
However, when I add ggplotly to add the interactive functionality, the markdown text in the title returns to just normal text:
mtcarsexp<-
mtcars |>
mutate(
cyl = as.factor(cyl)
) |>
ggplot(aes(x=mpg, y=hp, color=cyl)) +
geom_point(size=3) +
theme_bw() +
scale_color_manual(values=c("springgreen4", "darkorange3", "purple")) +
labs(
title = mt_title_text
) +
theme(
plot.title = ggtext::element_markdown(),
panel.grid.minor = element_blank(),
legend.position = 'none'
)
ggplotly(mtcarsexp)
It seems that perhaps I could use the concept in this question and, rather than use the title in the labs
, just create text, and position it in the place of the title, but I would much prefer to use the native labs
functionality.