Normal view
-
- A world where some currency loses value when handled would have a deflationary economy. What would the consequences to society be? – worldbuilding.stackexchange.comImagine a world which looks roughly like what we might call the “standard D&D setting”; not quite medieval, not quite Renaissance, something like 17th century London, where a penny buys a day’s ...
-
- A Vast New Data Set Could Supercharge the AI Hunt for Crypto Money LaunderingBlockchain analysis firm Elliptic, MIT, and IBM have released a new AI model—and the 200-million-transaction dataset it's trained on—that aims to spot the “shape” of bitcoin money laundering.
A Vast New Data Set Could Supercharge the AI Hunt for Crypto Money Laundering
-
- Converting micro amount into currencyI'm currently pulling report from Google Ad Manager API. One of the column im pulling is a revenue column which is a currency column. After I downloaded the data and save it into csv file, the revenue column return different than the value from the Google Ad manager website. Ive read that the google ad manager API return the currency value in micros. Is there any ways in the Google Ad Manager API that can directly change the value to currency value or do I need to create a python function to cha
Converting micro amount into currency
I'm currently pulling report from Google Ad Manager API. One of the column im pulling is a revenue column which is a currency column. After I downloaded the data and save it into csv file, the revenue column return different than the value from the Google Ad manager website. Ive read that the google ad manager API return the currency value in micros.
Is there any ways in the Google Ad Manager API that can directly change the value to currency value or do I need to create a python function to change it to the currency?
-
- Strange 'Currency Rates source not ready' forex_python errorI'm trying to understand forex API through python.The code that I am posting below worked for me on friday and I received all the conversion rates for the dates as desired. Strangely when I run the code today for some reason it says Currency Rates source not ready. Why is this happening? from forex_python.converter import CurrencyRates import pandas as pd c = CurrencyRates() from forex_python.converter import CurrencyRates c = CurrencyRates() df = pd.DataFrame(pd.date_range(start='8/16/2021
Strange 'Currency Rates source not ready' forex_python error
I'm trying to understand forex API through python.The code that I am posting below worked for me on friday and I received all the conversion rates for the dates as desired. Strangely when I run the code today for some reason it says
Currency Rates source not ready.
Why is this happening?
from forex_python.converter import CurrencyRates
import pandas as pd
c = CurrencyRates()
from forex_python.converter import CurrencyRates
c = CurrencyRates()
df = pd.DataFrame(pd.date_range(start='8/16/2021 10:00:00', end='8/22/2021 11:00:00', freq='600min'), columns=['DateTime'])
def get_rate(x):
try:
op = c.get_rate('CAD', 'USD', x)
except Exception as re:
print(re)
op=None
return op
df['Rate'] = df['DateTime'].apply(get_rate)
Currency Rates Source Not Ready
Currency Rates Source Not Ready
df
Out[17]:
DateTime Rate
0 2021-08-16 10:00:00 0.796374
1 2021-08-16 20:00:00 0.796374
2 2021-08-17 06:00:00 0.793031
3 2021-08-17 16:00:00 0.793031
4 2021-08-18 02:00:00 0.792469
5 2021-08-18 12:00:00 0.792469
6 2021-08-18 22:00:00 0.792469
7 2021-08-19 08:00:00 0.783967
8 2021-08-19 18:00:00 0.783967
9 2021-08-20 04:00:00 0.774504
10 2021-08-20 14:00:00 0.774504
11 2021-08-21 00:00:00 NaN
12 2021-08-21 10:00:00 NaN
13 2021-08-21 20:00:00 NaN
14 2021-08-22 06:00:00 NaN
How do I fix this issue? Is there a way to ignore NaN
while making calls itself? I feel that the API only gives results for Monday to Friday from 10 am to 5pm. So is there a way to just get those results.
-
- Users can concurrently withdraw above their wallet balance by initiating multiple concurrent withdrawalsI am updating a finance application built on node.js, mongodb and ```mongoose`` and I am currently facing a challenge with the logic to process fund withdrawals from the system. The problem is that a user can request an amount greater than their balance if they hit the fund withdrawal endpoint concurrently. For instance, with an available balance of $10, the user can process multiple $10 withdrawals as long as they do it concurrently. I know where the problem is coming from, just that I have bee
Users can concurrently withdraw above their wallet balance by initiating multiple concurrent withdrawals
I am updating a finance application built on node.js
, mongodb
and ```mongoose`` and I am currently facing a challenge with the logic to process fund withdrawals from the system. The problem is that a user can request an amount greater than their balance if they hit the fund withdrawal endpoint concurrently. For instance, with an available balance of $10, the user can process multiple $10 withdrawals as long as they do it concurrently.
I know where the problem is coming from, just that I have been unable to resolve it. I know this is happening because the actions are taking place concurrently so the user.balance
will always be 10
because the debit happens after the read. I am relatively new to backend development so pardon me if I don't use the correct terminologies. This is what my code looks like.
try{
// Perform tasks such as validating the account number, etc.
const user = await User.findById(req.user._id);
if(user.balance < req.body.amount)
return res.status(400).send({message:"Insufficient Balance"});
// update the user balance in the DB to user.balance - req.body.amount
return res.status(200).send({message:"Fund has been sent to the account provided"})
}
I tried an approach of updating the user record in the collection and using that response to check the user balance but I am not so sure if this will fix my issue completely or if there will still be loopholes or if there is a better way to handle this.
This is the second approach I tried out
try{
// Perform tasks such as validating the account number, etc.
const idx = uuid()
const user = await User.findByIdAndUpdate(req.user._id, {withdrawal_intent:idx} {
new: true,
});
if(user.balance < req.body.amount)
return res.status(400).send({message:"Insufficient Balance"});
// update the user balance in the DB to user.balance - req.body.amount
return res.status(200).send({message:"Fund has been sent to the account provided"})
}
I am open to remodelling the code to fit the best possible way to handle this
P.S: It's an old codebase so I might be using an old syntax of mongoose.
"mongoose": "^5.9.9"
-
- Guidance on Freqtrade Functions for Direct Buy and Sell Price SpecificationI'm relatively new to Freqtrade and would appreciate some guidance on the functions that would best suit my needs. I'm specifically interested in using Freqtrade's limit order mode without relying on indicators. Instead, I aim to directly provide buy and sell prices for the pairs I have, with the ability to dynamically adjust these prices in each iteration. Currently, I believe the bot operates at a frequency of approximately 1 minute. Could someone please clarify which functions I should focus
Guidance on Freqtrade Functions for Direct Buy and Sell Price Specification
I'm relatively new to Freqtrade and would appreciate some guidance on the functions that would best suit my needs.
I'm specifically interested in using Freqtrade's limit order mode without relying on indicators. Instead, I aim to directly provide buy and sell prices for the pairs I have, with the ability to dynamically adjust these prices in each iteration. Currently, I believe the bot operates at a frequency of approximately 1 minute.
Could someone please clarify which functions I should focus on for achieving this goal? From my understanding of the documentation, I'm considering the following functions:
populate_buy_trend() populate_sell_trend() custom_buy custom_sell custom_entry_price() custom_exit_price()
However, I'm unsure about the exact role of each function and which one(s) are most suitable for my requirements.
Any insights or examples illustrating the usage of these functions for direct buy and sell price specification would be greatly appreciated.
Thank you in advance for your assistance!
-
- Why doesn't Washington want to enact a law to punish all currency manipulators, including China? – politics.stackexchange.comSome policymakers and pundits have argued that currency manipulation–the effort to keep exports cheap by intervening in the foreign exchange market–is the most important issue international economic ...
Why doesn't Washington want to enact a law to punish all currency manipulators, including China? – politics.stackexchange.com
-
- I was approached to invest in short-term Blockchain node transactions. Is this scam? – money.stackexchange.comFull story: That person contacted me with these details : Short-term node transactions only occur when they occur, daily transactions can buy and sell coins at any time. Now more and more people are ...
I was approached to invest in short-term Blockchain node transactions. Is this scam? – money.stackexchange.com
-
- How to trigger a 2nd script to run simultaneously without affecting the Main script and with the possibility of adding multiple additional scripts?Note: I am updating my original post on 12/22/23 to better explain what is it that I am trying to achieve. Perhaps, this will make it easier to provide some suggestions. I am trying to build a notification bot! This bot will run continuously, checking every 3 min if a given item has restocked. If it has, it will send an email to a group of recipients to notify them about the restock. What I now want to do, is to split said group between a preferred group (which will be notified as soon as the bo
How to trigger a 2nd script to run simultaneously without affecting the Main script and with the possibility of adding multiple additional scripts?
Note: I am updating my original post on 12/22/23 to better explain what is it that I am trying to achieve. Perhaps, this will make it easier to provide some suggestions.
I am trying to build a notification bot! This bot will run continuously, checking every 3 min if a given item has restocked. If it has, it will send an email to a group of recipients to notify them about the restock. What I now want to do, is to split said group between a preferred group (which will be notified as soon as the bot finds the item back in stock) and a second group which will receive the same email but 10 min later (delayed notification).
The rest of my post still stands. Script 2 will wait 10 min and then send an email (which would take 2-5 seconds) and then exit. I do not need Script 2's result to run my main script. So I really do not need to keep track of Script 2 once it begins waiting.
As per some of the initial comments. At no point should there be more than 3 instances of Script 2 running simultaneously. Given that Script 2 waits for 10 min and then takes 3-5 seconds to complete its instructions, even if the Main Script finds the item in stock every 3 minutes, by the 4th time it finds the item (12 min since the first time), the first instance of Script 2 should have already ended. I hope that makes sense.
After reading about threading I came up with this super simple example of what I believe would be the structure of my scrip. Can someone tell me if this is wrong? Will I run out of resources if I run this script endlessly?
import threading
import time
import random
def delayed_email():
time.sleep(10)
print ("Delayed Email has been sent")
def immediate_email():
print ("Immediate Email has been sent")
while True:
number = random.randint(0,3)
print (number)
if number == 1:
immediate_email()
print ("Starting Script 2")
threading.Thread(target=delayed_email).start()
time.sleep(3)
----Original Post -----
I need help figuring out, conceptually, how to write a script that would do the following:
Main Script: Run continuously, checking every 3 minutes if a certain condition has been met. If condition has been met, then run some code and immediately trigger Script 2. After this, go back to sleeping for 3 minutes. Rinse and repeat either if the condition is met or not.
Script 2: If triggered by Main Script, if would sleep for 10 min and then run some code. Once finished, it is done. Main Script does not depend on this script. But Script 2 will only run if triggered by Main Script.
Questions about this: I do not think I can use async, in this scenario right? I believe this is more for concurrency, either multithread or multiprocessor, I just do not know which one and why. Or perhaps I'm wrong and I need to organize my logic some other way.
Also, Script 2 should be it's own entity that will live and die as soon as it finishes its code. This means that, for example:
- Main script runs and condition is met.
- Main script runs code and then triggers Script 2
- Main scrpit goes back to waiting for 3 minutes. While this is happening, Script 2 is sleeping for 10 minutes
- Main script's condition is met again 3 minutes later, hence it runs its code and immediately triggers a 2nd entity of Script 2 (since the first entity is still sleeping waiting for the initial 10 min to elapse)
In the example above, there are now two instances of Script 2 running simultaneously. One with 7 min left to sleep, while the new one is just starting to sleep 10 min, all while Main Script is going back to waiting for another 3 min.
With all this in mind, would multithread work or better to use multiprocessor? If none of this would work, what other options do I have to organize the logic to trigger Script 2 parallelly.
thanks
-
- Handling concurrency in LaravelI'm creating an API that interacts with a MySQL inventory database. We have 15 users that can reserve products, updating the database in the following way: Decreasing the on-hand value and increasing the reserved value of a product. The inventory table looks like this: id int sku varchar on-hand int reserved int The problem is: How to handle the update of the row if 2 users try to update it at the same time? The first aproach i was thinking about was using Transactions: <
Handling concurrency in Laravel
I'm creating an API that interacts with a MySQL inventory database. We have 15 users that can reserve products, updating the database in the following way:
- Decreasing the
on-hand
value and increasing thereserved
value of a product.
The inventory
table looks like this:
id int
sku varchar
on-hand int
reserved int
The problem is: How to handle the update of the row if 2 users try to update it at the same time?
The first aproach i was thinking about was using Transactions:
<?php
function reserveStock()
{
$db->beginTransaction();
// SELECT on-hand, reserved from inventory
// Update inventory values
$db->commit();
return response()->json([ 'success' => 1, 'data' => $data ])
}
The second one was using pessimist locking:
<?php
function reserveStock()
{
// SELECT on-hand, reserved from inventory with ->sharedLock()
// Update inventory values
return response()->json([ 'success' => 1, 'data' => $data ])
}
The third one was to create a updating
field with a value of cero. When selecting the products to update, i'd check the updating
field before doing anything with that rows. The problem i see here is that i'd have to loop the ones with updating != 0
until they become available. More selects and updates come fromt his aproach.
Which course of action if the best? There may be more options than the ones i've wrote here.
-
- Investing in Dogecoin: Price Prediction for 2023 and BeyondSource: Pixabay Dogecoin marks a decade of existence in 2023. A lot has happened since its inception, and the last few years were full of ups and downs. That goes for DOGE and the crypto market overall. It naturally begs the question — should you buy Dogecoin today? Our guide will remind you of the basics of the project, including the technologies underlying DOGE. We’ll discuss price history and present predictions from different sources regarding DOGE’s future cost. Meet First Meme Coi
Investing in Dogecoin: Price Prediction for 2023 and Beyond
Source: Pixabay
Dogecoin marks a decade of existence in 2023. A lot has happened since its inception, and the last few years were full of ups and downs. That goes for DOGE and the crypto market overall. It naturally begs the question — should you buy Dogecoin today?
Our guide will remind you of the basics of the project, including the technologies underlying DOGE. We’ll discuss price history and present predictions from different sources regarding DOGE’s future cost.
Meet First Meme Coin Project — Dogecoin
Dogecoin is a cryptocurrency that facilitates direct P2P payments. P2P stands for peer-to-peer, which indicates decentralization. Decentralized currencies are virtually the standard of the crypto industry and what attracts users to it.
But Dogecoin also had other characteristics to attract users. It’s that DOGE started as a joke! The coin’s logo features the Shiba Inu dog. The inspiration came from the famous Doge meme of the dog who speaks “weird” English.
Billy Markus and Jackson Palmer are Dogecoin creators. They specified that designing this token only took a few hours. Although it was imagined as a fun and light alternative to Bitcoin, DOGE served its purpose. People can use it for payments and trading via crypto exchanges.
A huge part of Dogecoin’s ongoing success is Elon Musk. He tweeted and discussed DOGE on multiple occasions, which often led to price boosts. Musk is even facing a lawsuit for running a pyramid scheme to support this currency.
DOGE doesn’t have a maximum supply. That’s another indicator that this is a parody currency. The creators wanted to send a message that there’s no point in digital token scarcity. Instead, adding new blocks to the chain generates new tokens. At this point, there are approximately 141.8 billion DOGE tokens in circulation. The project’s market cap is over $10 billion. That’s impressive and enough for Dogecoin to be in the top ten in the crypto rankings.
Technologies Underlying DOGE
Dogecoin shares many similarities with Bitcoin and Litecoin. The foundational one is that they both use blockchain technology. Dogecoin actually used Litecoin as the source code for its chain. That means DOGE uses a secure and distributed ledger that stores transaction details digitally. Thanks to cryptography, the records are immutable, and the network remains secure.
New DOGE coins become available via the mining process. That’s another similarity to BTC and LTC, with the exception there’s no maximum DOGE supply. Instead, a new block becomes available every minute. Miners receive 10,000 coins per block. If you do the math, that means 14.4 million DOGE tokens enter circulation daily.
Dogecoin uses the proof-of-work consensus mechanism. It means that miners put their computational power at the network’s disposal. The devices solve complicated math tasks to confirm and store info about each transaction on the blockchain. The reward comes in the form of DOGE coins.
Dogecoin Price Retrospective
Source: Pixabay
The crypto market is very volatile, so going too far back when analyzing prices might not be worth the hassle. However, a quick reminder couldn’t hurt. DOGE entered the market in December 2013. It was worth $0.0002047 at the time. It took only three days to gain a 500% value boost.
Some struggles followed, and DOGE hit an all-time low in 2015. The lowest value was $0.00008547. As the crypto industry progressed, Dogecoin’s price increased. Its all-time high occurred in 2021, when it was worth $0.7376.
How Did Dogecoin Perform in 2022?
It’s now time to dig into the recent price history of Dogecoin. DOGE was worth $0.173 at the start of January 2022. It was a rough year for the entire crypto. That makes it no wonder that DOGE’s price also went down.
Dogecoin dropped to $0.13 in February and $0.11 in March. The following month was encouraging, and the worth went up to $0.172 in April. That was only short-term because DOGE continued dropping. In June, it reached $0.05.
Only short price boosts followed, but the final few months were somewhat better, and DOGE finished the year at $0.07.
DOGE Price Prediction
The current value of DOGE is $0.074. It’s hard to expect the token’s worth will change significantly before the year ends. Predictors mostly agree although some indicate that DOGE could go up to $0.098.
For 2024, Dogecoin forecasts vary. The majority believes it will reach at least $0.096, while some put it at $0.14. Those most optimistic even put DOGE’s maximum for 2024 at $0.28. The truth is that it might be a bit too generous unless the entire market flourishes in the coming months.
DOGE could be worth $0.16 in 2025, with several predictors placing it at $0.20 or higher. The prognosis goes up to $0.39, and some even place it at $0.45 in 2026. More realistic forecasts for this year place DOGE anywhere from $0.25 to $0.35.
If we switch our focus to long-term forecasts, we notice that Dogecoin could be worth anywhere from $0.37 to $0.73. It’s harder to tell how much DOGE would be worth at the end of the decade. Most experts agree that the token should go at least $0.5 by 2030. Some predictors even believe Dogecoin could cross the $1 threshold.
It Is Never Too Late to Buy DOGE
The critical consideration for Dogecoin is that it’s inflationary. It means there’s no maximum supply, and there’s no halving to increase the scarcity. New DOGE tokens become available daily. That affects DOGE’s attractiveness for long-term investment. In theory, maintaining the current token’s value is actually a success since more coins keep entering circulation.
The current strategy, however, makes Dogecoin worth considering. You can always use it in short-term or daily trading on Paybis. It’s also smart to follow the news since DOGE is highly influenced by the related events. We witnessed that with Elon Musk’s tweets, which improved the coin’s value almost every time. One thing is certain — it’s never too late to buy DOGE. That’s why it deserves a place on your monitoring list, and if you deem it suitable for your portfolio, then also in your crypto wallet.
The post Investing in Dogecoin: Price Prediction for 2023 and Beyond appeared first on Productivity Land.
-
- How do central banks outside the U.S. issue USD? – politics.stackexchange.comHow do central banks outside the U.S. issue USD? Sometimes, during a recession, you need to issue currency to get the economy going, but sometimes you have a central bank that uses the currency of ...
How do central banks outside the U.S. issue USD? – politics.stackexchange.com
-
- Options Trading For Startup Founders: A Strategic ApproachOptions trading is a sophisticated financial product that may accomplish many investing objectives. Options trading may be a helpful tool for company founders for hedging against market volatility, obtaining funding, creating income, and limiting risk. However, it is vital to highlight that options trading is dangerous, and company owners must grasp the dangers before participating in this activity. This blog article will review several typical options trading tactics and the strategic
Options Trading For Startup Founders: A Strategic Approach
Options trading is a sophisticated financial product that may accomplish many investing objectives. Options trading may be a helpful tool for company founders for hedging against market volatility, obtaining funding, creating income, and limiting risk.
However, it is vital to highlight that options trading is dangerous, and company owners must grasp the dangers before participating in this activity.
This blog article will review several typical options trading tactics and the strategic applications of options trading for business owners. We’ll also provide you with some pointers on how to get started with options trading.
We invite you to read on, whether you are a seasoned investor or a total newbie, to discover more about how options trading might help your firm.
Options Trading And Startup Founders
Options trading is a way to bet on the future price of stocks or other assets. It’s like making a bet with a friend, but instead of betting on sports, you’re betting on whether a stock’s price will go up or down.
Options trading matters to startup founders because it can help them manage their money wisely. Startups often need cash to grow, and options can be a way to protect that cash or even make more of it. Think of it as a financial tool that can give you more control over your startup’s future. With options, you can limit how much money you might lose. It’s like having a safety net for your investments.
Understanding options trading is a valuable skill for startup founders. It’s about using financial tools to protect and grow your startup’s money. In the next sections, we’ll explore different options trading strategies and how to manage the risks so that you can make smart choices for your startup’s financial success.
Strategic Uses Of Options Trading For Startup Founders
Options trading can be a valuable tool for startup founders, offering strategic ways to navigate the financial landscape. Here are some key uses:
Hedging Against Market Volatility
Startup entrepreneurs are often subjected to extreme market volatility. This is because startups often produce new and creative goods and services, which might face market uncertainty. Options trading allows startup owners to lock in a price for their underlying asset, such as their company’s shares or the stock of a key supplier, enabling them to hedge against market volatility.
A startup entrepreneur, for example, may buy put options on their company’s shares to protect themselves against a drop in the stock price. If the stock price falls, the founder may sell their shares at the striking price, the price stated in the option contract, by exercising their put options.
Raising Capital
Options trading may also be utilized to obtain funds for new businesses. A startup entrepreneur, for example, may sell covered calls on their company’s shares. This would provide the call option buyer the right, but not the responsibility, to purchase the founder’s shares at a defined price on or before a given date. If the stock price increases over the strike price, the buyer of the call options will almost certainly exercise their options, forcing the founders to sell their shares. If the stock price does not increase over the strike price, the founder will keep their shares and get the premium paid by the call option buyer.
Generating Income
Options trading may also be utilized to create revenue for new businesses. A company entrepreneur, for example, may sell options on a crucial supplier’s shares. The buyer of the put options would, therefore, have the right, but not the responsibility, to sell the founder shares of the supplier’s stock at a set price on or before a given date. If the stock price goes below the strike price, the put option buyer will most likely exercise their options, requiring the founder to purchase the shares. If the stock price does not fall below the strike price, the founder will keep the premium the put option buyer paid.
Mitigating Risk
Startups may also utilize options trading to reduce risk. A company entrepreneur, for example, may buy defensive puts on a crucial customer’s shares. This would allow the founder the right, but not the responsibility, to sell the customer’s stock shares at a certain price on or before a defined date. If the client’s stock price falls, the founder may execute their put options and sell their shares at the strike price. The founder would be protected against losses on their investment in the customer’s shares.
Understanding that options trading is a sophisticated and dangerous activity is critical. Before investing in options trading, startup entrepreneurs should carefully assess the dangers involved. It is also critical to check with a financial professional to verify that options trading is acceptable for their specific situation.
Options Trading Strategies for Startup Founders
Options trading offers various strategies for startup founders to manage risk and optimize their financial positions. Here are some essential options trading strategies simplified for you:
1. Covered Calls
Covered calls are a good way for startup founders to generate income from their existing stock holdings. Imagine you have a favorite gadget and are willing to sell it but don’t want to let it go completely. Covered calls are like renting out that gadget. You own the stock and are willing to sell it at a specific price (the strike price) in the future.
By selling covered calls, founders can receive a premium payment, a non-refundable deposit from the buyer of the call option. If the stock price rises above the strike price, the call option buyer will exercise their option and purchase the founder’s shares. However, the founder will keep the premium payment even if the stock price does not rise above the strike price.
Covered calls can be adapted to crypto trading, allowing you to generate income by selling call options against your cryptocurrency holdings. Additionally, some crypto trading bots like Bitcoin Trader can automate the process of executing covered call strategies, making it more convenient and efficient for crypto investors.
2. Protective Puts
Protective puts are a good way for startup founders to hedge against downside risk. By buying protective puts, founders can set a price floor for their stock holdings. If the stock price falls below the put option’s strike price, the founder can exercise their option and sell their shares at the higher strike price. This can help to protect founders from large losses if the stock price declines.
Picture your startup as a valuable possession. You want to protect it from unexpected accidents. Protective puts are like getting insurance for your startup. You buy put options to set a price floor for your stock. If the stock price falls, you can sell it at the higher put option price, preventing big losses.
3. Bullish and Bearish Spreads
Bullish and bearish spreads can be used to profit from a directional move in the stock market. Bullish spreads are used when a trader is optimistic about the stock market’s future direction, while bearish spreads are used when a trader is pessimistic. Spreads can limit risk while allowing for the potential to generate profits.
Sometimes, you have a hunch about the market’s direction. You can use call spreads if you’re optimistic (bullish) about a stock or index. If you’re pessimistic (bearish), you can use put spreads. These strategies allow you to profit from your market outlook while limiting potential losses.
4. Long and Short Straddles
Long and short straddles are strategies that can be used to profit from a big move in the stock market, regardless of the direction of the move. Long straddles involve buying both a call and a put option with the same strike price and expiration date. Short straddles involve selling both a call and a put option with the same strike price and expiration date. Both straddle strategies can be profitable if the stock moves in either direction. However, it is important to note that straddle strategies are also more risky than other options trading strategies.
Imagine you’re in a quiet room, expecting a surprise but not knowing if it’ll be good or bad. Long straddles are like preparing for any surprise.
5. Iron Condors
Iron condors are strategies that can be used to profit from a range-bound stock market. Iron condors involve selling both a call spread and a put spread with different strike prices. As long as the stock price stays within a certain range, the seller of the iron condor will profit. However, if the stock price moves outside of the range, the seller of the iron condor will experience losses.
Think of the stock market as a range-bound playground. Iron condors are like playing the middle ground. You simultaneously sell a call spread and a put spread with different strike prices. You can profit if the stock stays within a certain range.
6. Butterfly Spreads
Butterfly spreads are more complex options trading strategies that can be used to profit from a specific price move in the stock market. Butterfly spreads involve buying and selling multiple options with different strike prices. The risk-reward profile of a butterfly spread will vary depending on the specific strikes and quantities of options used. However, butterfly spreads can generate profits if the stock price stays close to a certain price.
Butterfly spreads are like making a bet on a butterfly landing on a particular flower. You buy and sell multiple options with different strike prices, creating a risk-reward profile that profits if the stock stays close to a certain price.
Final Thoughts
Before participating in options trading, startup founders should carefully assess the risks involved and speak with a financial expert to verify that options trading is acceptable for their circumstances.
It may be used to hedge against risk, produce revenue, and reduce risk. However, it is crucial to realize that option trading is equally dangerous. Several options trading techniques are accessible, and your circumstances and objectives will determine your optimal approach. Before implementing any plan, it is critical to analyze and comprehend its risks and benefits.
Before you begin trading options, you need to develop a trading strategy. Your trading strategy should include objectives, risk tolerance, and trading guidelines.
If you are a company entrepreneur considering adopting options trading, I recommend doing homework and consulting with a financial adviser. Options trading may be a powerful instrument, but it must be used cautiously.
The post Options Trading For Startup Founders: A Strategic Approach appeared first on Productivity Land.
-
- Understanding Bitcoin and Vesper Finance’s Hold and Grow Approaches In the ever-evolving landscape of cryptocurrencies, the last decade has witnessed a remarkable surge in both growth and innovation. Notably, the matrixator.io has emerged as a central player in this digital financial revolution, shaping the way we perceive and interact with the world of digital assets. Complementing Bitcoin’s skyrocketing prominence, decentralized finance (DeFi) platforms like Vesper Finance have also risen to prominence, introducing investors to novel avenues for nurturing thei
Understanding Bitcoin and Vesper Finance’s Hold and Grow Approaches
In the ever-evolving landscape of cryptocurrencies, the last decade has witnessed a remarkable surge in both growth and innovation. Notably, the matrixator.io has emerged as a central player in this digital financial revolution, shaping the way we perceive and interact with the world of digital assets. Complementing Bitcoin’s skyrocketing prominence, decentralized finance (DeFi) platforms like Vesper Finance have also risen to prominence, introducing investors to novel avenues for nurturing their digital wealth. In the following article, we will embark on an in-depth exploration of the fascinating realms of Bitcoin and Vesper Finance. Our journey will involve dissecting their respective “Hold and Grow” strategies, meticulously scrutinizing their strengths and vulnerabilities, and gaining a comprehensive understanding of their significance in the crypto ecosystem.
Bitcoin: A Digital Gold Standard
Bitcoin’s Genesis and Key Characteristics
Bitcoin, created in 2009 by an anonymous entity known as Satoshi Nakamoto, is often referred to as “digital gold” due to its scarcity and store of value properties. It operates on a decentralized network of computers and is built on a technology called blockchain.
Store of Value vs. Medium of Exchange
Bitcoin’s primary function has evolved over time. Initially conceived as a peer-to-peer electronic cash system, it has now become more of a store of value, akin to gold, with many investors holding it as a long-term asset.
The Role of Scarcity in Bitcoin’s Value
One of the fundamental factors driving Bitcoin’s value is its fixed supply. With a maximum supply capped at 21 million coins, scarcity is built into the protocol. This scarcity has led to a perception of Bitcoin as “digital gold” and a hedge against inflation.
Historical Price Trends and Market Sentiment
Bitcoin’s price history has been marked by extreme volatility, with notable bull and bear markets. Market sentiment, investor adoption, and macroeconomic factors have all influenced its price movements.
Vesper Finance: A DeFi Platform for Crypto Enthusiasts
Introduction to Decentralized Finance (DeFi)
DeFi refers to a set of financial services and applications built on blockchain technology. These services aim to decentralize and democratize traditional financial functions, such as lending, borrowing, and trading.
Vesper Finance’s Mission and Features
Vesper Finance is a DeFi platform designed to simplify and optimize the process of earning yield on cryptocurrency holdings. It offers a range of user-friendly features and tools for crypto enthusiasts.
The Vesper Approach to Yield Generation
Vesper employs a dynamic strategy that involves yield farming and liquidity provision on various DeFi protocols. It seeks to maximize returns while managing risks through sophisticated algorithms and automation.
Vesper’s Community and Governance
Vesper is governed by its community of users, who have a say in platform improvements and decisions. This decentralized governance model allows Vesper to adapt and evolve with the changing DeFi landscape.
Hold and Grow Strategies
The Traditional Approach: HODLing Bitcoin
Historical Success Stories
HODLing, a misspelling of “hold,” is a strategy where Bitcoin investors buy and hold their assets over the long term. This approach has yielded substantial gains for early adopters.
Risks and Challenges
However, HODLing is not without risks. Bitcoin’s price can be highly volatile, and investors may need to weather significant price fluctuations.
Vesper Finance’s Yield-Focused Approach
Yield Farming and Liquidity Provision
Vesper’s approach involves actively participating in DeFi protocols to generate yield. This includes strategies like yield farming and providing liquidity to decentralized exchanges.
Risk Management and Diversification
Vesper employs risk management protocols to mitigate potential losses, and it offers a range of pools with varying levels of risk and return potential.
Earning Passive Income with Vesper
Users can earn passive income by depositing their assets into Vesper’s pools and allowing the platform to manage their investments.
Comparing Bitcoin Holding and Vesper’s Strategies
Risk vs. Reward: A Comparative Analysis
When comparing the two strategies, it’s essential to weigh the potential rewards against the associated risks.
Long-Term vs. Short-Term Perspective
Bitcoin holding typically involves a long-term investment horizon, while Vesper’s strategies may offer more frequent opportunities for yield generation.
Liquidity and Flexibility Considerations
Bitcoin is highly liquid and can be easily traded, whereas Vesper’s strategies may involve locking up funds for a specific period.
Real-World Use Cases and Success Stories
Case Studies of Successful Bitcoin Investors
Examining real-life examples of individuals who held Bitcoin for an extended period and achieved financial success.
Vesper Finance Users’ Experiences and Results
Insights into the experiences and returns of individuals using Vesper Finance to grow their cryptocurrency holdings.
The Future of Bitcoin and Vesper Finance
Bitcoin’s Role in the Future Financial Landscape
Speculations about Bitcoin’s potential role in the traditional financial system and as a global reserve asset.
The Evolution of DeFi and Vesper’s Growth Prospects
Considerations regarding the ongoing evolution of DeFi and Vesper’s position within this dynamic ecosystem.
Regulatory and Security Concerns
Discussing the regulatory challenges and security considerations that both Bitcoin and DeFi platforms like Vesper face.
Conclusion
In conclusion, this in-depth exploration of Bitcoin and Vesper Finance’s “Hold and Grow” strategies reveals the diverse landscape of cryptocurrency investment. Bitcoin, hailed as digital gold, offers a secure store of value with its limited supply, while Vesper Finance represents the dynamic DeFi realm, providing opportunities for active asset growth. The comparison between these approaches underscores the importance of risk assessment, investment horizon, and liquidity considerations. Real-world examples highlight the potential rewards of both strategies. As Bitcoin integrates into traditional finance and DeFi continues to evolve, regulatory and security challenges remain crucial. Ultimately, the choice between Bitcoin and Vesper Finance, or a combination of both, should align with individual goals and risk tolerance. In this ever-changing crypto landscape, staying informed and making informed investment decisions remain paramount.
The post Understanding Bitcoin and Vesper Finance’s Hold and Grow Approaches appeared first on Productivity Land.
-
- Concurrent calls to Phoenix using phoenixdb python packageI am currently stress testing an API that relies on the phoenixdb Python package to execute select queries on a Phoenix view. However, it appears that the calls made using phoenixdb are synchronous in nature, causing requests to be answered in a synchronous manner. Currently, I only found a workaround by encapsulating the phoenixdb call within a dedicated event loop, like this : import asyncio from phoenixdb import connect from fastapi import FastAPI app = FastAPI() # This takes around 5 secon
Concurrent calls to Phoenix using phoenixdb python package
I am currently stress testing an API that relies on the phoenixdb Python package to execute select queries on a Phoenix view. However, it appears that the calls made using phoenixdb are synchronous in nature, causing requests to be answered in a synchronous manner.
Currently, I only found a workaround by encapsulating the phoenixdb call within a dedicated event loop, like this :
import asyncio
from phoenixdb import connect
from fastapi import FastAPI
app = FastAPI()
# This takes around 5 seconds
def synchronous_phoenixdb_query():
database_url = 'http://localhost:8765/'
connection = connect(database_url, autocommit=True)
cursor = connection.cursor()
# Execute a query
cursor.execute('SELECT * FROM my_table')
result = cursor.fetchall()
cursor.close()
connection.close()
return result
# Asynchronous wrapper
async def asynchronous_phoenixdb_query():
loop = asyncio.get_event_loop()
result = await loop.run_in_executor(None, synchronous_phoenixdb_query)
return result
@app.get("/phoenixdb_query")
async def phoenixdb_query():
result = await asynchronous_phoenixdb_query()
return {"result": result}
However I am not sure about the limits of this approach.
-
- What Cryptos Should You Get Amidst the NFT Slump? One of the biggest stories in the blockchain world currently is about NFTs. Specifically, it is about how 95% of them have lost their value. NFTs have had a good run in the consumer market and blockchain space, but experts now say that the bubble has completely burst. But with NFTs now deemed as not a worthwhile investment, blockchain investors have to look elsewhere to maximize returns. The good news is that there is a plethora of cryptos that are likely to fill this gap and offer returns.
What Cryptos Should You Get Amidst the NFT Slump?
One of the biggest stories in the blockchain world currently is about NFTs. Specifically, it is about how 95% of them have lost their value. NFTs have had a good run in the consumer market and blockchain space, but experts now say that the bubble has completely burst.
But with NFTs now deemed as not a worthwhile investment, blockchain investors have to look elsewhere to maximize returns. The good news is that there is a plethora of cryptos that are likely to fill this gap and offer returns.
Anarchy
One of the more eye-catching of these opportunities is Anarchy, a meme token that aims to take on the current unfair financial system. Those who buy into it can see their investment soar through several token value increase strategies that the project has in place. All these are done through its native $ANA token, and while the project is called Anarchy, it is actually very democratic.
All users get voting rights within the system and can choose both ambassadors who will handle the treasury on a day-to-day basis and the projects that the community will collectively invest in. The project is currently counting down to its ICO, after which those who have indicated interest will receive their tokens.
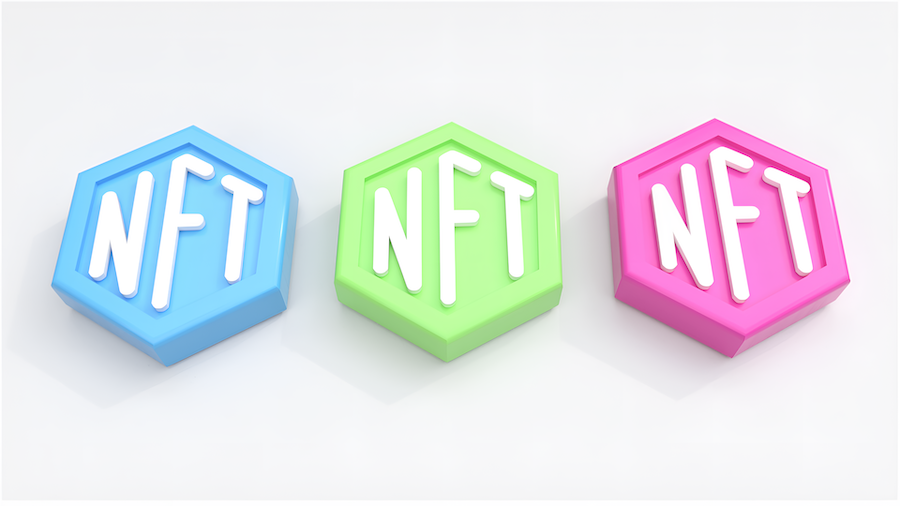
BEP-20
Then there’s Bitcoin BSC, a green BEP-20 version of Bitcoin based on the Binance Smart Chain. The idea behind this token is to take Bitcoin investors ‘back in time’ to when Bitcoin was sold for $0.99 back in 2011. Users can stake this token to earn rewards as an effective trading strategy, and these BTCBSC tokens are paid out every 10 minutes. The token is available on several DEXs, and there are plans to offer investors a long-term staking opportunity they wouldn’t get with the original Bitcoin. Overall, Bitcoin BSC is offering users the best of both worlds.
ERC-20
A few years ago, online communities rocked the financial world with the Gamestop saga. Now, a crypto project called Wall Street Bets Memes (WSM) pays homage to it. Its native token, an ERC-20 token on the Ethereum blockchain, can be staked for rewards and is backed by a community with over a million members. But it is more than just an investment scheme it is also a way of organizing against the monopoly that Wall Street has on the financial world. It has raised over $25 million in its presale alone and seems very promising.
If you plan to buy when the market is low, now’s the time
The current NFT slump represents the loss of a major investment vehicle for blockchain lovers and potentially the death of an entire sub-industry. On the upside, the crypto industry seems more than capable of providing alternatives in the form of the tokens detailed above. From meme tokens, which are a homage to a critical point in online financial communities, to those that give a spin on an iconic crypto to those trying to buck the system, the options are limitless. This means that for investors, there will always be something to buy into.
The post What Cryptos Should You Get Amidst the NFT Slump? appeared first on Productivity Land.
-
- Update value in ConcurrentDictionary by conditionI have class: public class LazyConcurrentDictionary<TKey, TValue> { private readonly ConcurrentDictionary<TKey, Lazy<TValue>> _concurrentDictionary; public LazyConcurrentDictionary() { _concurrentDictionary = new ConcurrentDictionary<TKey, Lazy<TValue>>(); } public TValue GetOrAdd(TKey key, Func<TKey, TValue> valueFactory) { var lazyResult = _concurrentDictionary.GetOrAdd(key, k => new Lazy<TValue>(
Update value in ConcurrentDictionary by condition
I have class:
public class LazyConcurrentDictionary<TKey, TValue>
{
private readonly ConcurrentDictionary<TKey, Lazy<TValue>> _concurrentDictionary;
public LazyConcurrentDictionary()
{
_concurrentDictionary = new ConcurrentDictionary<TKey, Lazy<TValue>>();
}
public TValue GetOrAdd(TKey key, Func<TKey, TValue> valueFactory)
{
var lazyResult = _concurrentDictionary.GetOrAdd(key,
k => new Lazy<TValue>(() => valueFactory(k), LazyThreadSafetyMode.ExecutionAndPublication));
return lazyResult.Value;
}
}
I want use this class for manage token. A token expired in 2 hours.
var dictionary = new LazyConcurrentDictionary<string, Lazy<Tuple<string, DateTime>>>();
var result = dictionary.GetOrAdd("80", k => new Lazy<Tuple<string, DateTime>>(() =>
{
return new Tuple<string, DateTime>(Guid.NewGuid().ToString(), DateTime.Now);
}));
For example for key 80 have value tuple with token and datetime now.
How do change code when time expired get new token?
best regards
-
- Can you initiate a lawsuit against Russia in the United States to recover seized assets? – law.stackexchange.comEver since the start of the Ukraine conflict in 2022, Russia has halted payments to international creditors and individuals holding assets like stocks. Is it possible for an average person who happens ...
Can you initiate a lawsuit against Russia in the United States to recover seized assets? – law.stackexchange.com
-
- Rust Thread Within Struct that Mutates selfI am trying to implement the raft consensus algorithm using Rust. First I am trying to implement some sort of local message exchange between 2 servers. This is my server struct: pub struct Server { my_id: u8, senders: Vec<(u8, Sender<String>)>, receivers: Vec<Mutex<Receiver<String>>>, message_queue: Vec<String>, request_message_queue: Vec<String>, running: bool, } I am failing in writing a thread that receives the messages sent b
Rust Thread Within Struct that Mutates self
I am trying to implement the raft consensus algorithm using Rust. First I am trying to implement some sort of local message exchange between 2 servers.
This is my server struct:
pub struct Server {
my_id: u8,
senders: Vec<(u8, Sender<String>)>,
receivers: Vec<Mutex<Receiver<String>>>,
message_queue: Vec<String>,
request_message_queue: Vec<String>,
running: bool,
}
I am failing in writing a thread that receives the messages sent by the senders.
pub fn send_message(&self, to: u8, message: String) {
self.senders.iter().for_each(|(id, sender)| {
if to == *id {
sender.send(message.clone()).unwrap();
}
});
}
pub fn init(&'static mut self) {
self.running = true;
thread::scope(move |scope| {
scope.spawn(move |scope| {
self.receivers.iter().for_each(|rec| {
let r = rec.lock().unwrap();
if !r.is_empty() {
r.recv().unwrap();
}
drop(r)
});
});
})
.expect("HEY");
}
With the code above I keep getting this error after calling the init method in the main.
server1.lock().unwrap().init();
| ^^^^^^^^^^^^^^----------------
| |
| borrowed value does not live long enough
| argument requires that `server1` is borrowed for `'static`
...
24 | }
| - `server1` dropped here while still borrowed
I also believe that my main function is far for good. Should I be using this many mutexes?
fn main() {
let mut server1 = Arc::new(Mutex::new(Server::new(0)));
let mut server2 = Arc::new(Mutex::new(Server::new(1)));
let mut server3 = Arc::new(Mutex::new(Server::new(2)));
server1.lock().unwrap().init();
server1.lock().unwrap().connect(server2.clone());
server1.lock().unwrap().connect(server3.clone());
server1
.lock()
.unwrap()
.send_message(1, "HELLO WORLD".to_string());
server1
.lock()
.unwrap()
.send_message(1, "HELLO WORLD".to_string());
server2.lock().unwrap().receive();
}
Could some one please explain how can this be done. The idea is that I want to be able to send and receive the messages at the same time.